Raisim Server¶
RaisimServer serializes raisim::World
and streams the data to clients via tcp/ip.
We provide the raisimUnity (doc) client, which visualizes a raisim::World
.
The basic usage is described in the doc.
Other than just visualizing a raisim::World
, raisim::RaisimServer
can visualize additional visual objects.
An example can be found here.
The example will be displayed as following
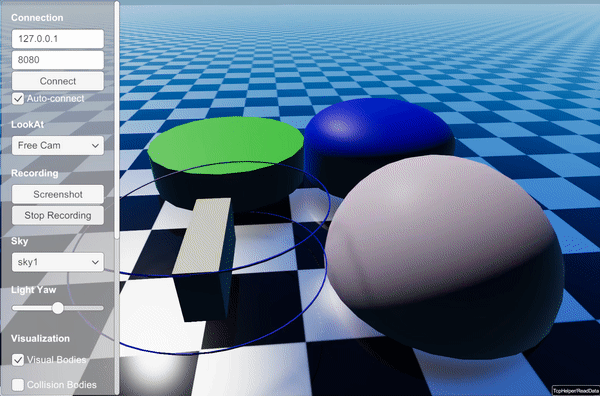
RaisimServer API¶
-
class
RaisimServer
¶ Public Functions
-
inline explicit
RaisimServer
(World *world)¶ - Parameters
world – [in] the world to visualize. create a raisimSever for a world.
-
inline void
setupSocket
(int port = 8080)¶ - Parameters
port – [in] the port number to open the socket Setup the port so that it can accept (acceptConnection) incoming connections
-
inline void
acceptConnection
(int seconds)¶ - Parameters
seconds – [in] the number of seconds to wait for a connection from a client Accept a connection to the socket. Only one client can be connected at a time
-
inline void
closeConnection
() const¶ Close the current connection from a client. For new connections, the port has to be setup again.
-
inline void
setMap
(const std::string &map)¶ - Parameters
map – [in] name of the map to be loaded. This only works with RaisimUnreal. Currently, the following maps are available “”: Empty map. Supports weather and time. “simple”: Empty map. Simple sky for faster rendering. “wheat”: a flat wheat field “dune”: flat dune
-
inline void
launchServer
(int port = 8080)¶ - Parameters
port – [in] port number to stream start spinning.
-
inline void
integrateWorldThreadSafe
()¶ set the world mutex. This will prevent visualization thread reading from the world (otherwise, there can be a segfault). Integrate the world.
-
inline void
applyInteractionForce
()¶ Apply interaction force, which is specified by the user in the visualizer (raisimUnreal). This is automatically called in raisim::RaisimServer::integrateWorldThreadSafe.
-
inline void
hibernate
()¶ hibernate the server. This will stop the server spinning.
-
inline void
wakeup
()¶ wake up the server. Restart the server from hibernation
-
inline void
killServer
()¶ stop spinning the server and disconnect the client
-
inline void
lockVisualizationServerMutex
()¶ lock the visualization mutex so that the server cannot read from the world
-
inline void
unlockVisualizationServerMutex
()¶ unlock the visualization mutex so that the server can read from the world
-
inline bool
isTerminateRequested
()¶ - Returns
boolean representing if the termination requested
-
inline ArticulatedSystemVisual *
addVisualArticulatedSystem
(const std::string &name, const std::string &urdfFile, double colorR = 0, double colorG = 0, double colorB = 0, double colorA = 0)¶ - Parameters
name – [in] the name of the visual articulated system object
urdfFile – [in] the path to the urdf file
colorR – [in] the red value of the color (max=1)
colorG – [in] the green value of the color (max=1)
colorB – [in] the blue value of the color (max=1)
colorA – [in] the alpha value of the color (max=1)
- Returns
the articulated system visual pointer add an articulated system without physics
-
inline void
removeVisualArticulatedSystem
(ArticulatedSystemVisual *as)¶ - Parameters
as – [in] ArticulatedSystemVisual to be removed remove a visualized articulated system
-
inline InstancedVisuals *
addInstancedVisuals
(const std::string &name, Shape::Type type, const Vec<3> &size, const Vec<4> &color1, const Vec<4> &color2)¶ - Parameters
name –
type –
size –
color1 –
color2 –
- Returns
-
inline Visuals *
addVisualSphere
(const std::string &name, double radius, double colorR = 1, double colorG = 1, double colorB = 1, double colorA = 1, const std::string &material = "", bool glow = false, bool shadow = false)¶ - Parameters
name – [in] the name of the visual object
radius – [in] radius of the sphere
colorR – [in] the red value of the color (max=1)
colorG – [in] the green value of the color (max=1)
colorB – [in] the blue value of the color (max=1)
colorA – [in] the alpha value of the color (max=1)
material – [in] visualization material
glow – [in] to glow or not (not supported)
shadow – [in] to cast shadow or not (not supported)
- Returns
the sphere pointer add a sphere without physics
-
inline Visuals *
addVisualBox
(const std::string &name, double xLength, double yLength, double zLength, double colorR = 1, double colorG = 1, double colorB = 1, double colorA = 1, const std::string &material = "", bool glow = false, bool shadow = false)¶ - Parameters
name – [in] the name of the visual object
xLength – [in] length of the box
yLength – [in] width of the box
zLength – [in] height of the box
colorR – [in] the red value of the color (max=1)
colorG – [in] the green value of the color (max=1)
colorB – [in] the blue value of the color (max=1)
colorA – [in] the alpha value of the color (max=1)
material – [in] visualization material
glow – [in] to glow or not (not supported)
shadow – [in] to cast shadow or not (not supported)
- Returns
the box pointer add a box without physics
-
inline Visuals *
addVisualCylinder
(const std::string &name, double radius, double length, double colorR = 1, double colorG = 1, double colorB = 1, double colorA = 1, const std::string &material = "", bool glow = false, bool shadow = false)¶ - Parameters
name – [in] the name of the visual object
radius – [in] radius of the cylinder
length – [in] length of the cylinder
colorR – [in] the red value of the color (max=1)
colorG – [in] the green value of the color (max=1)
colorB – [in] the blue value of the color (max=1)
colorA – [in] the alpha value of the color (max=1)
material – [in] visualization material
glow – [in] to glow or not (not supported)
shadow – [in] to cast shadow or not (not supported)
- Returns
the cylinder pointer add a cylinder without physics
-
inline Visuals *
addVisualCapsule
(const std::string &name, double radius, double length, double colorR = 1, double colorG = 1, double colorB = 1, double colorA = 1, const std::string &material = "", bool glow = false, bool shadow = false)¶ - Parameters
name – [in] the name of the visual object
radius – [in] radius of the capsule
length – [in] length of the capsule
colorR – [in] the red value of the color (max=1)
colorG – [in] the green value of the color (max=1)
colorB – [in] the blue value of the color (max=1)
colorA – [in] the alpha value of the color (max=1)
material – [in] visualization material
glow – [in] to glow or not (not supported)
shadow – [in] to cast shadow or not (not supported)
- Returns
the capsule pointer add a capsule without physics
-
inline Visuals *
addVisualMesh
(const std::string &name, const std::string &file, const Vec<3> &scale = {1, 1, 1}, double colorR = 0, double colorG = 0, double colorB = 0, double colorA = -1, bool glow = false, bool shadow = false)¶ - Parameters
name – [in] the name of the visual mesh object
file – [in] file name of the mesh
scale – [in] scale of the mesh
colorR – [in] the red value of the color (max=1)
colorG – [in] the green value of the color (max=1)
colorB – [in] the blue value of the color (max=1)
colorA – [in] the alpha value of the color (max=1). Ignore color when negative
glow – [in] to glow or not (not supported)
shadow – [in] to cast shadow or not (not supported)
- Returns
the mesh visual pointer add a mesh without physics
-
inline VisualMesh *
addVisualMesh
(const std::string &name, const std::vector<float> &vertexArray, const std::vector<uint8_t> &colorArray, const std::vector<int32_t> &indexArray, double colorR = 0, double colorG = 0, double colorB = 0, double colorA = 1, bool glow = false, bool shadow = false)¶ - Parameters
name – [in] the name of the visual mesh object
file – [in] file name of the mesh
vertexArray – array of vertices Should be a multiple of 3
colorArray – array of colors in RGB. Should be a multiple of 3
indexArray – array of triangle index
colorR – red color value (if colorArray is empty)
colorG – green color value (if colorArray is empty)
colorB – blue color value (if colorArray is empty)
colorA – alpha color value (if colorArray is empty)
glow – to glow or not (not supported)
shadow – to cast shadow or not (not supported)
- Returns
the mesh visual pointer (VisualMesh struct)
-
inline Visuals *
addVisualArrow
(const std::string &name, double radius, double height, double colorR = 0, double colorG = 0, double colorB = 0, double colorA = -1, bool glow = false, bool shadow = false)¶ - Parameters
name – [in] the name of the visual mesh object
radius – [in] radius of the arrow
height – [in] height of the arrow
colorR – [in] the red value of the color (max=1)
colorG – [in] the green value of the color (max=1)
colorB – [in] the blue value of the color (max=1)
colorA – [in] the alpha value of the color (max=1)
glow – [in] to glow or not (not supported)
shadow – [in] to cast shadow or not (not supported)
- Returns
the visual pointer add an arrow without physics
-
inline HeightMapVisual *
addVisualHeightMap
(const std::string &name, const std::string &fileName, double centerX, double centerY, double colorR = 0, double colorG = 0, double colorB = 0, double colorA = -1)¶ - Parameters
name – [in] the name of the visual heightmap object
fileName – [in] the raisim text file which will be used to create the height map
centerX – [in] x coordinate of the center of the height map
centerY – [in] y coordinate of the center of the height map
colorR – [in] the red value of the color (max=1)
colorG – [in] the green value of the color (max=1)
colorB – [in] the blue value of the color (max=1)
colorA – [in] the alpha value of the color (max=1)
- Returns
pointer to the created visual height map
-
inline HeightMapVisual *
addVisualHeightMap
(const std::string &name, const std::string &pngFileName, double centerX, double centerY, double xScale, double yScale, double heightScale, double heightOffset, double colorR = 0, double colorG = 0, double colorB = 0, double colorA = -1)¶ - Parameters
name – [in] the name of the visual heightmap object
pngFileName – [in] the png file which will be used to create the height map
centerX – [in] x coordinate of the center of the height map
centerY – [in] y coordinate of the center of the height map
xSize – [in] x width of the height map
ySize – [in] y length of the height map
heightScale – [in] a png file (if 8-bit) has pixel values from 0 to 255. This parameter scales the pixel values to the actual height
heightOffset – [in] height of the 0-value pixel
colorR – [in] the red value of the color (max=1)
colorG – [in] the green value of the color (max=1)
colorB – [in] the blue value of the color (max=1)
colorA – [in] the alpha value of the color (max=1)
- Returns
pointer to the created visual height map
-
inline HeightMapVisual *
addVisualHeightMap
(const std::string &name, double centerX, double centerY, TerrainProperties &terrainProperties, double colorR = 0, double colorG = 0, double colorB = 0, double colorA = -1)¶ - Parameters
name – [in] the name of the visual heightmap object
centerX – [in] x coordinate of the center of the height map
centerY – [in] y coordinate of the center of the height map
terrainProperties – [in] perlin noise parameters which will be used to create the height map
colorR – [in] the red value of the color (max=1)
colorG – [in] the green value of the color (max=1)
colorB – [in] the blue value of the color (max=1)
colorA – [in] the alpha value of the color (max=1)
- Returns
pointer to the created visual height map
-
inline HeightMapVisual *
addVisualHeightMap
(const std::string &name, size_t xSamples, size_t ysamples, double xSize, double ySize, double centerX, double centerY, const std::vector<double> &height, double colorR = 0, double colorG = 0, double colorB = 0, double colorA = -1)¶ - Parameters
name – [in] the name of the visual heightmap object
xSamples – [in] how many points along x axis
ySamples – [in] how many points along y axis
xSize – [in] x width of the height map
ySize – [in] y length of the height map
centerX – [in] x coordinate of the center of the height map
centerY – [in] y coordinate of the center of the height map
height – [in] a vector of doubles representing heights. the size should be xSample X ySamples
colorR – [in] the red value of the color (max=1)
colorG – [in] the green value of the color (max=1)
colorB – [in] the blue value of the color (max=1)
colorA – [in] the alpha value of the color (max=1)
- Returns
pointer to the created visual height map
-
inline HeightMapVisual *
addVisualHeightMap
(const std::string &name, const HeightMap *hm, double colorR = 0, double colorG = 0, double colorB = 0, double colorA = -1)¶ - Parameters
name – [in] the name of the visual heightmap object
heightmapToBeCloned – [in] Another height map to be cloned
colorR – [in] the red value of the color (max=1)
colorG – [in] the green value of the color (max=1)
colorB – [in] the blue value of the color (max=1)
colorA – [in] the alpha value of the color (max=1)
- Returns
pointer to the created height map
-
inline void
removeVisualHeightMap
(HeightMapVisual *hm)¶ - Parameters
hm – [in] ArticulatedSystemVisual to be removed remove a visualized articulated system
-
inline PolyLine *
addVisualPolyLine
(const std::string &name)¶ - Parameters
name – [in] the name of the polyline
- Returns
the polyline pointer add a polyline without physics
-
inline PolyLine *
getVisualPolyLine
(const std::string &name)¶ - Parameters
name – [in] the name of the polyline get visualized polyline
-
inline ArticulatedSystemVisual *
getVisualArticulatedSystem
(const std::string &name)¶ - Parameters
name – [in] the name of the visual articulated system get visualized articulated system
-
inline HeightMapVisual *
getVisualHeightMap
(const std::string &name)¶ - Parameters
name – [in] the name of the visual articulated system get visualized height map
-
inline void
removeVisualPolyLine
(const std::string &name)¶ - Parameters
name – [in] the name of the polyline to be removed remove an existing polyline
-
inline Visuals *
getVisualObject
(const std::string &name)¶ - Parameters
name – [in] the name of the visual object to be retrieved
- Returns
visual object with a specified name retrieve a visual object with a specified name
-
inline void
removeVisualObject
(const std::string &name)¶ - Parameters
name – [in] the name of the visual object to be removed remove an existing visual object
-
inline void
startRecordingVideo
(const std::string &videoName)¶ - Parameters
videoName – [in] name of the video file to be saved. The videoName must be a valid file name (e.g., no spaces, ending in .mp4) start recording video. RaisimUnity only supports video recording in linux
-
inline void
stopRecordingVideo
()¶ stop recording video
-
inline void
setCameraPositionAndLookAt
(const Eigen::Vector3d &pos, const Eigen::Vector3d &lookAt)¶ - Parameters
pos – [in] the position of the camera
lookAt – [in] the forward direction of the camera (the up direction is always z-axis) set the camera to a specified position
-
inline void
focusOn
(raisim::Object *obj)¶ - Parameters
obj – [in] the object to look at move the camera to look at the specified object
-
inline bool
isConnected
() const¶ - Returns
if a client is connected to a server check if a client is connected to a server
-
inline bool
waitForMessageFromClient
(int seconds)¶ - Parameters
seconds – [in] the number of seconds to wait for the client
- Returns
true if there is a message from the client This method checks if there is a message from the client. It waits a specified time for a message. If this method is not used, the application will stop if there is no message.
-
inline bool
needsSensorUpdate
()¶ Check if there is any sensor that has to be updated from the visualizer
- Returns
if any of the sensors needs an update
-
inline bool
processRequests
()¶ Synchronous update method. Receive a request from the client, process it and return the requested data to the client. The method return false if 1) the client failed to respond 2) the client protocol version is different 3) the client refused to receive the data 4) the client did not send the sensor data in time
- Returns
if succeeded or not.
-
inline bool
waitForNewClients
(int seconds)¶ wait for a new client
- Parameters
seconds – how long to wait for a new client
- Returns
if a new client was found or not
-
inline void
requestSaveScreenshot
()¶ Saves the screenshot (the directory is chosen by the visualizer)
-
inline TimeSeriesGraph *
addTimeSeriesGraph
(std::string title, std::vector<std::string> names, std::string xAxis, std::string yAxis)¶ Only works with RaisimUnreal. Please read the “atlas” example to see how it works.
- Parameters
title – [in] title of the chart
names – [in] name of the data curves to be plotted
xAxis – [in] title of the x-axis
yAxis – [in] title of the y-axis
- Returns
pointer to the created Time Series Graph
-
inline BarChart *
addBarChart
(std::string title, std::vector<std::string> names)¶ Only works with RaisimUnreal. Please read the “atlas” example to see how it works.
- Parameters
title – [in] title of the chart
names – [in] name of the data histogram to be plotted
- Returns
pointer to the created Bar Chart
-
inline explicit
Visuals API¶
-
struct
Visuals
¶ Subclassed by raisim::VisualMesh
Public Functions
-
inline void
setSphereSize
(double radius)¶ - Parameters
radius – [in] the raidus of the sphere. set size of the sphere.
-
inline void
setBoxSize
(double x, double y, double z)¶ - Parameters
x – [in] length.
y – [in] width.
z – [in] height. set size of the box.
-
inline void
setCylinderSize
(double radius, double height)¶ - Parameters
radius – [in] the raidus of the cylinder.
height – [in] the height of the cylinder. set size of the cylinder.
-
inline void
setCapsuleSize
(double radius, double height)¶ - Parameters
radius – [in] the raidus of the capsule.
height – [in] the height of the capsule. set size of the capsule.
-
inline void
setPosition
(double x, double y, double z)¶ - Parameters
x – [in] x coordinate of the visual object.
y – [in] y coordinate of the visual object.
z – [in] z coordinate of the visual object. set the position of the visual object.
-
inline void
setOrientation
(double w, double x, double y, double z)¶ - Parameters
w – [in] angle part of the quaternion.
x – [in] scaled x coordinate of the rotation axis.
y – [in] scaled y coordinate of the rotation axis.
z – [in] scaled z coordinate of the rotation axis. set the orientation of the visual object.
-
inline void
setPosition
(const Eigen::Vector3d &pos)¶ - Parameters
pos – [in] position of the visual object in Eigen::Vector3d. set the position of the visual object.
-
inline void
setOrientation
(const Eigen::Vector4d &ori)¶ - Parameters
ori – [in] quaternion of the visual object in Eigen::Vector4d. set the orientation of the visual object.
-
inline void
setColor
(double r, double g, double b, double a)¶ - Parameters
r – [in] red value of the color (max=1).
g – [in] green value of the color (max=1).
b – [in] blue value of the color (max=1).
a – [in] alpha value of the color (max=1). set the color of the visual object.
-
inline Eigen::Vector3d
getPosition
()¶ - Returns
the position of the visual object. get the position of the visual object.
-
inline Eigen::Vector4d
getOrientation
()¶ - Returns
the orientation of the visual object. get the orientation of the visual object.
-
inline void
lockMutex
()¶ locks visualObject mutex. This can be used if you use raisim in a multi-threaded environment.
-
inline void
unlockMutex
()¶ unlock visualObject mutex. This can be used if you use raisim in a multi-threaded environment.
-
inline void
Polyline API¶
-
struct
PolyLine
¶ Public Functions
-
inline void
setColor
(double r, double g, double b, double a)¶ - Parameters
r – [in] red value of the color (max=1).
g – [in] green value of the color (max=1).
b – [in] blue value of the color (max=1).
a – [in] alpha value of the color (max=1). set the color of the polyline.
-
inline void
addPoint
(const Eigen::Vector3d &point)¶ - Parameters
point – [in] new polyline point. append a new point to the polyline.
-
inline void
clearPoints
()¶ clear all polyline points.
-
inline void
lockMutex
()¶ locks polyLine mutex. This can be used if you use raisim in a multi-threaded environment.
-
inline void
unlockMutex
()¶ unlock polyLine mutex. This can be used if you use raisim in a multi-threaded environment.
-
inline void
ArticulatedSystemVisual API¶
-
struct
ArticulatedSystemVisual
¶ Public Functions
-
inline void
setColor
(double r, double g, double b, double a)¶ - Parameters
r – [in] red value (max=1)
g – [in] green value (max=1)
b – [in] blue value (max=1)
a – [in] alpha value (max=1) set color. if the alpha value is 0, it uses the original color defined in the mesh file
-
inline void
setGeneralizedCoordinate
(const Eigen::VectorXd &gc)¶ - Parameters
gc – [in] the generalized coordinate set the configuration of the visualized articulated system
-
inline void
lockMutex
()¶ locks polyline mutex. This can be used if you use raisim in a multi-threaded environment.
-
inline void
unlockMutex
()¶ unlock polyline mutex. This can be used if you use raisim in a multi-threaded environment.
-
inline void